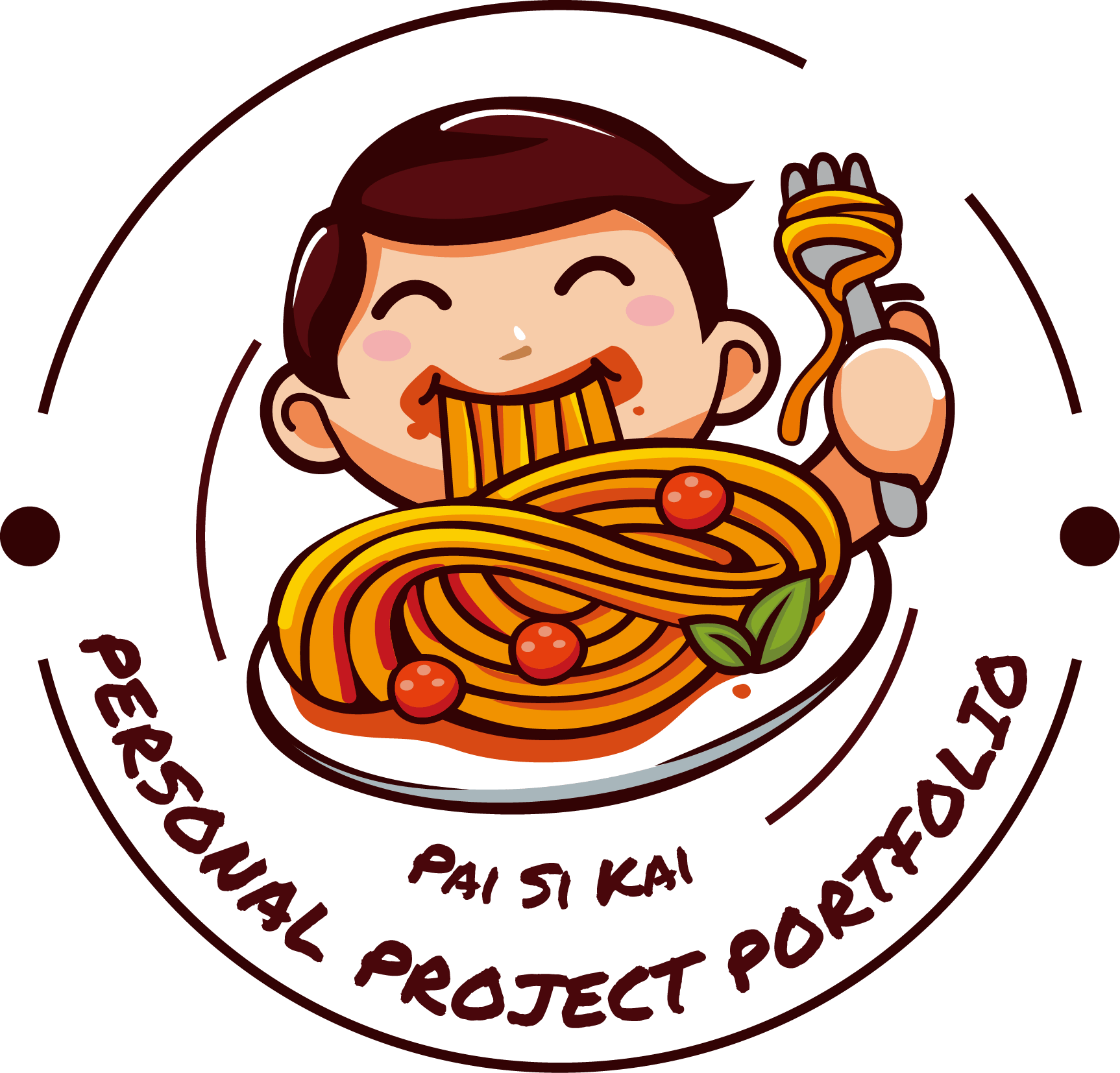
Pai Si Kai - Project Portfolio for The Food Diary
Overview
Welcome to my Personal Project Portfolio! This is a documentation of my contributions to the development of my group’s application, The Food Diary, as part of a software engineering module (CS2103T) in the National University of Singapore. My role in this project was mainly to implement the functionality of reviews for each restaurant.
The Food Diary is a desktop application that allows users to store their personal food reviews and obtain food recommendations. It is written in Java and is meant for people who prefer to work with a Command Line Interface (CLI), an example being people who type rapidly. It also gives users the benefit of having a clear and interactive Graphical User Interface (GUI) created with JavaFX.
Summary of contributions
-
Core development: I added the core functionality of adding, editing and deleting reviews
-
This feature is essential to the purpose of the application as it is the only way for users to store their personal review entries into the application. Users are also prone to mistakes when typing or a change in opinion. Hence, the ability to edit or delete previously created reviews will prove useful.
-
-
Enhancement: I considered the needs to users in a rush and implemented default reviews. This allows users to quickly add a review with a shortened and simpler command than the traditional addReview command. Default reviews that are pre-configured are added so that users do not have to face the hassle of manually entering every field needed.
-
Code contributed: Here is a link to my code on the Project Code Dashboard (Reposense).
-
Other contributions:
-
Project management:
-
Managed release
v1.2.1
(mid v1.3) andv1.3
on GitHub
-
-
Enhancements to existing features:
-
Created additional utility classes and methods for greater testability of code (Pull request #73), from which other members of the team wrote test code.
-
-
Documentation:
-
Modified and added our details and pictures into the About Us page: (Pull request #3)
-
Updated our Contact Us page (same PR as above)
-
-
Community:
-
Contributions to the User Guide
This section details my contributions to the User Guide pertaining to the functionality of Reviews. They showcase my ability to write documentation targeting end-users. |
Review Features
Adding a review: addReview
Adds a review to the Food Diary
Format: addReview INDEX re/ENTRY rr/RATING
Examples:
-
addReview 2 re/Peach Pie was amazing rr/4
Adds a review to the second restaurant in the list.
Editing a specified review : editReview
Edit selected fields in a specified entry.
Format: editReview INDEX [re/ENTRY] [rr/RATING]
Steps to execute editReview
command:
-
From the main screen of the application, first select a restaurant by clicking on it with the mouse or using the
select INDEX
command.
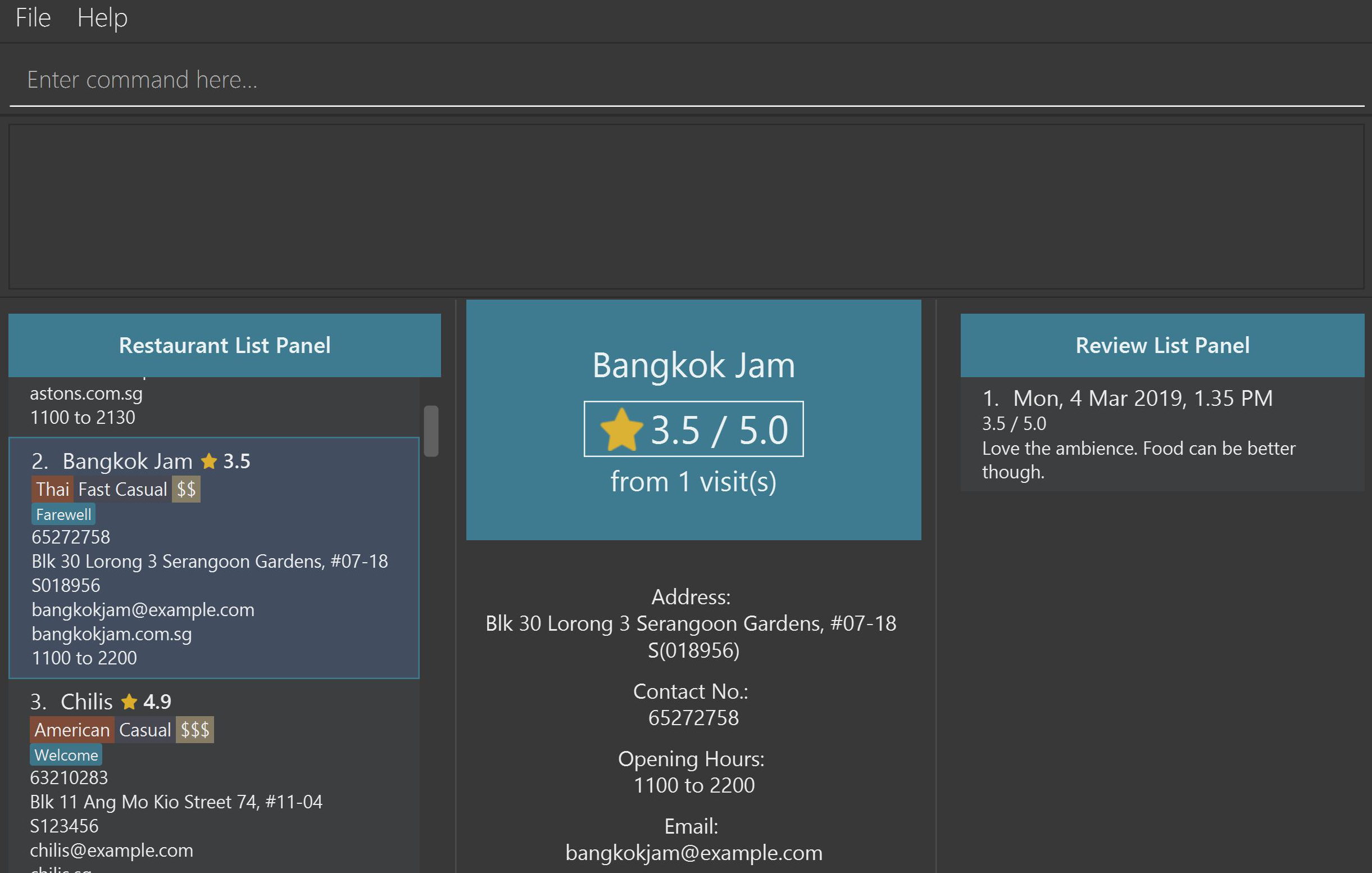
Figure 1. Upon selecting a restaurant, the reviews of the restaurant will show on the rightmost review panel
-
Following the above, enter the command. Upon successful execution of the command:
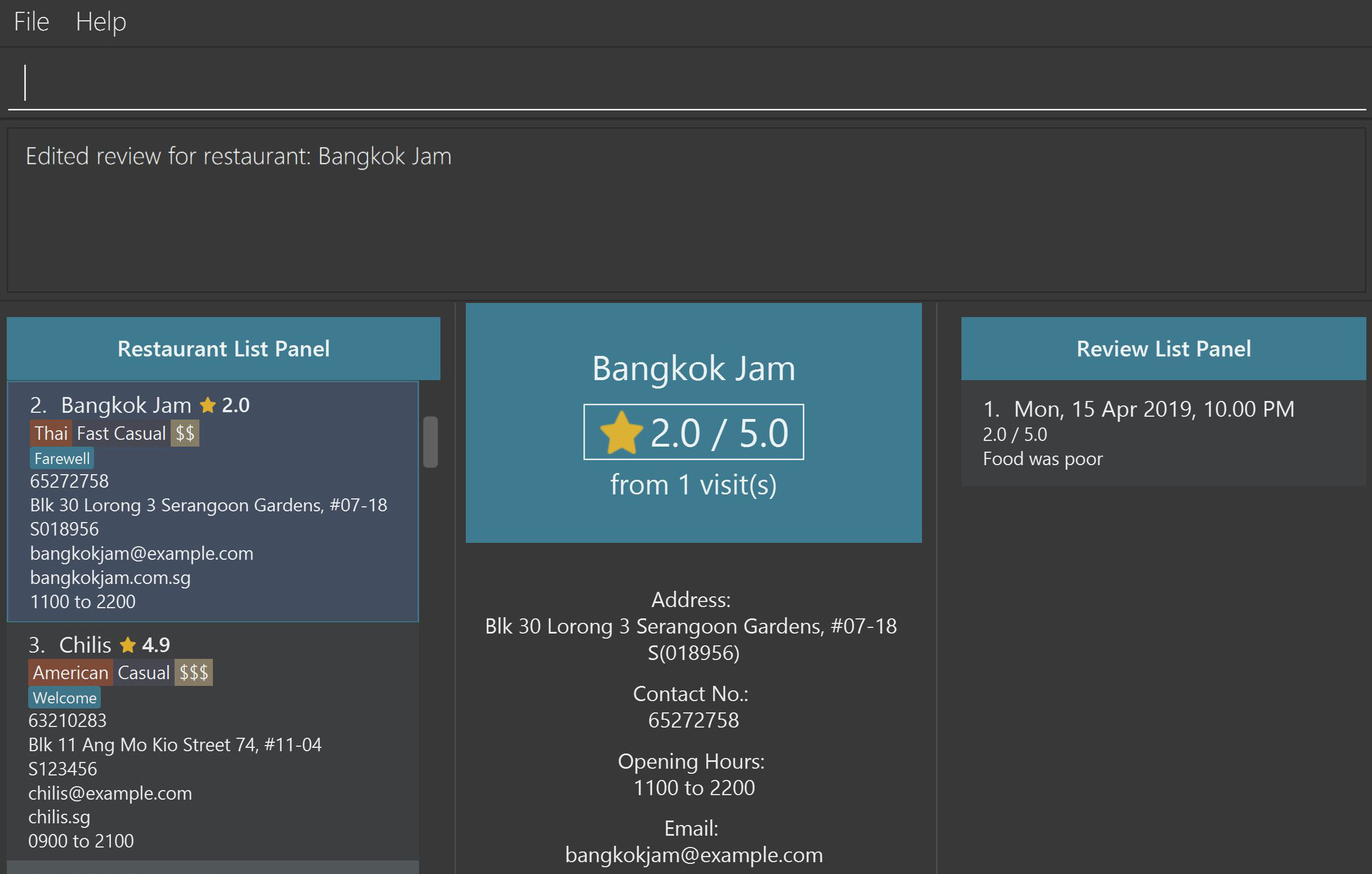
Figure 2. The above screenshot shows the end-product of the successful execution of editReview
command
Examples:
-
editReview 2 re/Food isn’t the best
Edits the comment of the second review toFood isn’t the best
-
editReview 2 re/Food isn’t the best rr/4
Edits the comment of the second review toFood isn’t the best
and the rating to be4
.
Deleting a review : deleteReview
Deletes the review from the Food Diary.
Format: deleteReview INDEX
Examples:
-
deleteReview 2
Deletes the 2nd review of the selected restaurant in the Food Diary.
Adding a default review : addR
In a real hurry? Fear not because The Food Diary has built in default reviews! You can use this command to quickly add a review with minimal typing. The command and syntax to add default reviews is much shorter and simpler than the normal addReview
command.
These default reviews are representative of the rating scale from 1 - 5. There is a default review entry associated with each default review rating. They range from:
-
Default review of rating 1: "Very poor, never ever go again." to
-
Default review of rating 5: "Excellent, must go again."
Format: addR INDEX NUMBER
Examples:
-
addR 1 4
adds a review of rating 4 and entry "Good, would go again." to the first restaurant on the displayed list.
Edit default review entry (Coming in v2.0)
Edits the default review entry according to the specified index. This allows you to customize your default review entries.
Format: editR NUMBER re/NEW_ENTRY
Examples:
-
editR 4 re/Not too shabby at all
changes the default review corresponding to rating 4 to "Not too shabby at all".
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Add/AddDefault/Edit/Delete Reviews
Reviews are a core feature of the application. Each review is specific to a restaurant, and represents one visit/experience at that particular restaurant. This section describes the implementation of the functions dealing with creating, modifying and modifying reviews. There is also an enhancement in the form of adding default reviews which will be elaborated on.
Add Reviews
This command adds a review to a restaurant specified by the INDEX
argument.
Command format: addReview INDEX re/(ENTRY) rr/(RATING)
Current Implementation
The functionality of the addReview command can be better understood with the following activity diagram:
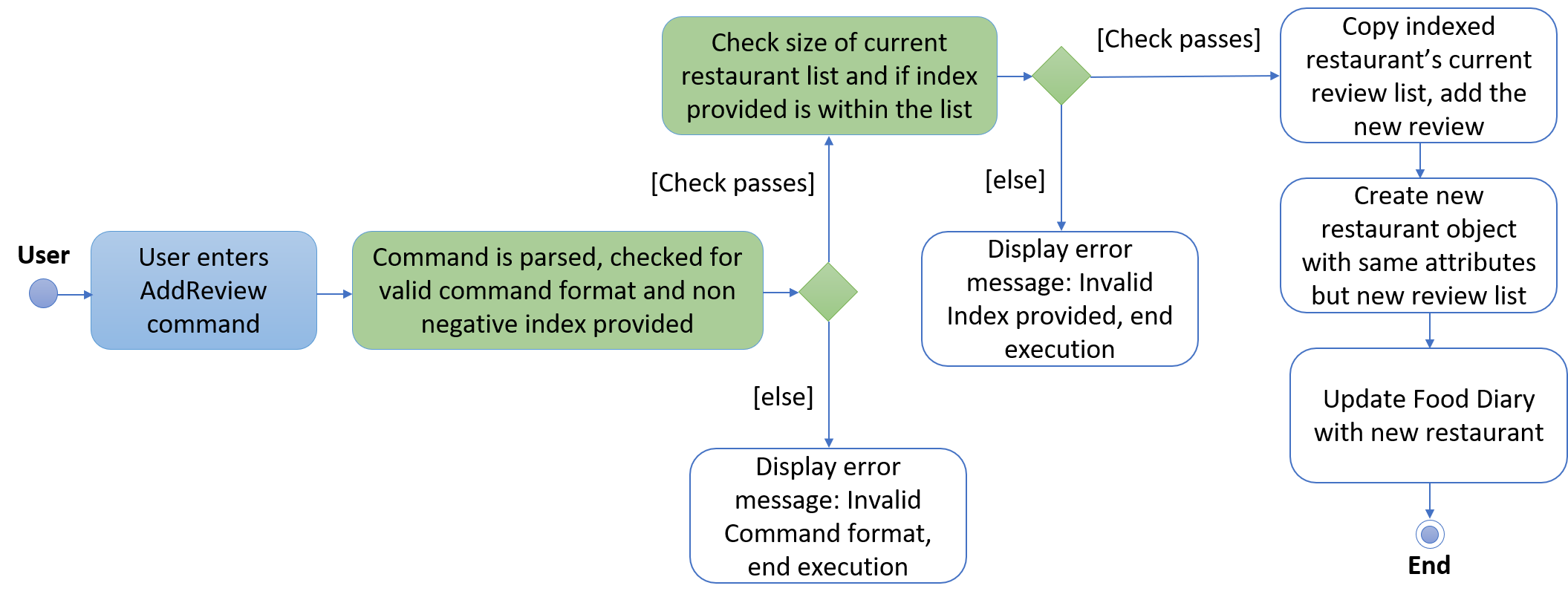
The execution of this command involves:
Step 1. Retrieving the last shown list from the Model
and retrieving the restaurant indicated by the index from the last shown list.
Step 2. Creating a new list of reviews that copies everything from the original restaurant’s list of reviews and inserting the new review into the list and creating a new restaurant object with the new list.
Step 3. Replacing the original restaurant with the new restaurant object in the model.
Refer to the sequence diagram below for an illustration of how this command is executed.
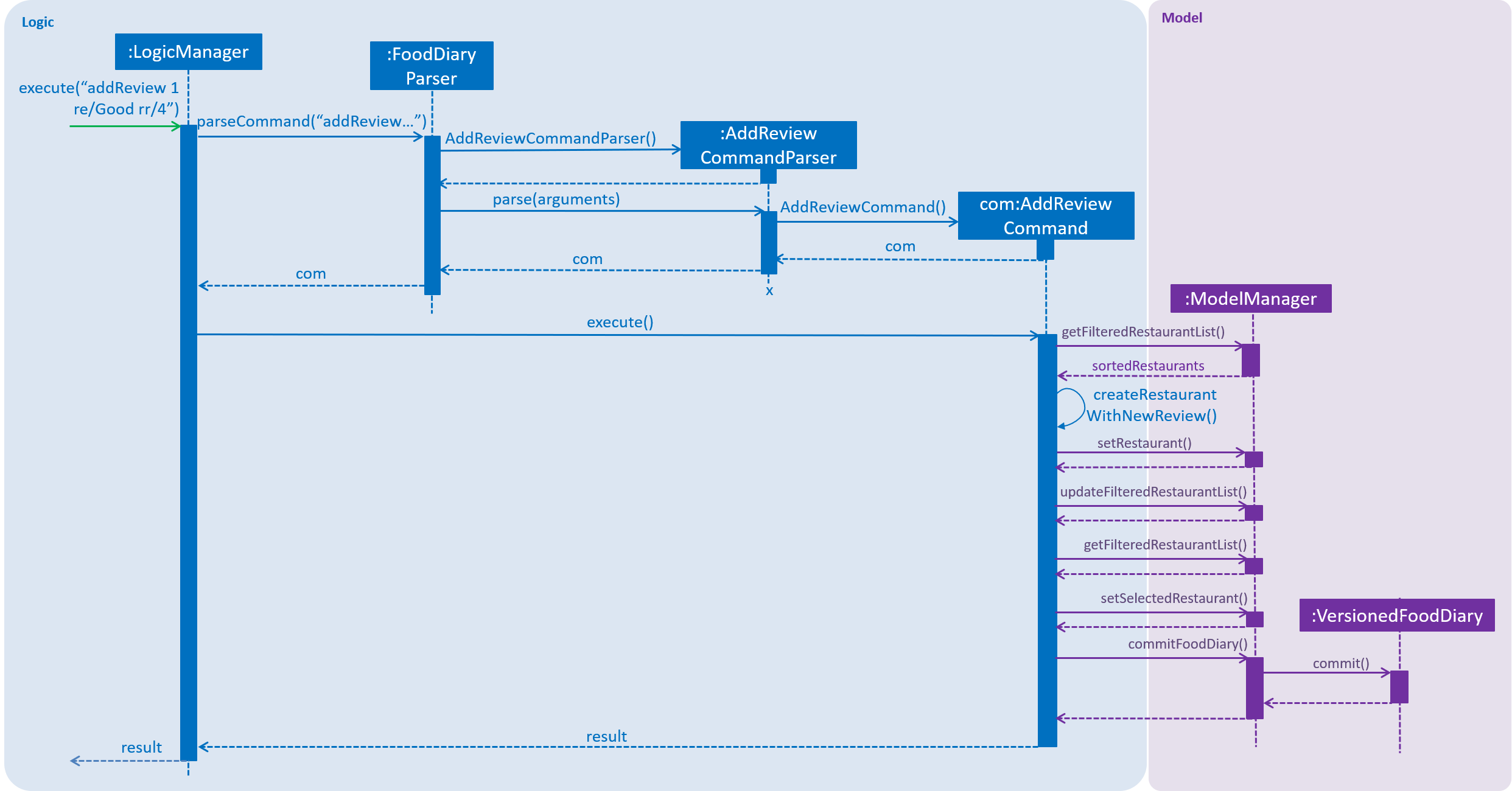
Design considerations
Plausible alternative implementations to the functionality are discussed in this section to illustrate the thought process and rationale behind our design decisions. You may wish to consider these ideas when further developing this application.
-
Alternative 1: Add reviews directly to the same restaurant object’s list rather than creating a new restaurant by object copy
Pros |
Less expensive operation to simply modify the same objects list of reviews |
Cons |
Requires modification of the |
AddDefault Reviews
This is a command to add pre-defined reviews with a shorter and simpler syntax. It is especially useful for people in a rush and who do not wish to enter the whole review.
Users need only specify the INDEX
of the restaurant they want to add the review to, and a NUMBER
representing the default review they wish to leave. For example, "addR 1 2" will add the default review of rating 2 to the 1st restaurant on the list.
Command format: addR INDEX NUMBER
Current Implementation
The Food Diary currently supports 5 default reviews, each corresponding to a rating from 1-5 inclusive. Do have a look at the User Guide for these 5 entries!
The review Entry
, which is composed of a String value, will be taken from the AddDefaultReviewUtil
class. The review Rating
used is the indicated NUMBER
in the command. A new Review
object is created from these two attributes and added.
Design Considerations
This alternative was considered as well:
-
Alternative 1: Adding a review directly from the new
AddDefaultReviewCommand
instead of creating anAddReviewCommand
However, this violates the DRY (Don’t Repeat Yourself) Principle, as the code to add a review will be repeated. |
Edit Reviews
This command allows the modification of exiting reviews in the Food Diary.
Command format: editReview INDEX [re/ENTRY] [rr/RATING]
, where INDEX
refers to the index of the review to edit in the selected restaurant, ENTRY
and RATING
are optional, but at least one of the two fields must be specified.
Current Implementation
Do refer to the sequence diagram below for a clearer picture of the implementation of this command. Note that the interaction between the EditReviewCommand
and the Model
is left out as it is the same as the one in AddReviewCommand
above.
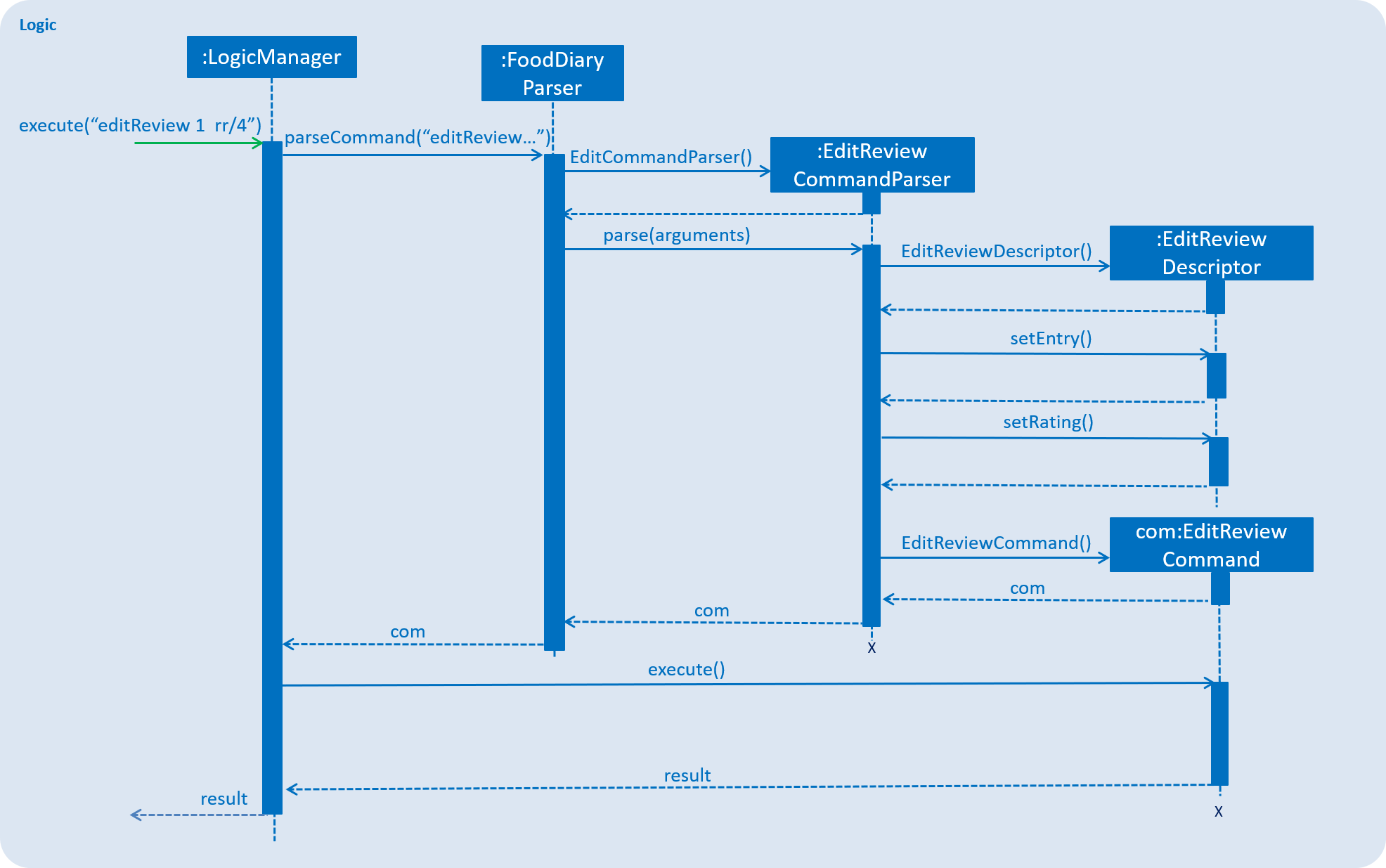
The components involved in the execution of this command include the FoodDiaryParser
, the EditReviewCommandParser
and the EditReviewCommand
class itself. The main difference from the AddReviewCommand
is the EditReviewDescriptor
class, which is used to store the changes for the review.
The EditReviewDescriptor
class can contain optional fields. This is so that the command does not have to modify every field in a review and users can specify which field they would like to modify.
The steps involved in the execution of this command are:
-
Step 1: Creating the
EditReviewDescriptor
based on input arguments. -
Step 2: Retrieving the currently selected restaurant from the model.
-
Step 3: Creating a new restaurant based on the currently selected restaurant and the
EditReviewDescriptor
-
Step 4: Replacing the selected restaurant with the new restaurant object in the model.
Design Considerations
-
Alternative 1: Have users input the index of the restaurant into the command instead of having to select a restaurant first
Considering the technical aspect, there is not much of a difference between the two implementations. However, this design decision took into account the fact that users most likely had to select the restaurant to look at the review they wish to edit before editing it. As such, it reduces the arguments input by the user, and makes use of what is likely going to happen anyways (the selection of the restaurant). |